Declaration
procedure SetPoints(Points: array of TPoint; PointBase: TIEPointBase = iepbRange); overload;
procedure SetPoints(Index: Integer; X, Y: Integer; PointBase: TIEPointBase = iepbRange; SnapAngles: Boolean = False); overload;
Description
Sets the three points used to define the angle (where point 1 is vertex).
Parameter | Description |
Points | An array of three TPoint values |
Index/X/Y | An index of a TPoint (0 - 2) and its X and Y value |
PointBase | What base units point values are specified in |
SnapAngles | Set to true to adjust the angle to ensure it aligns with LayersRotateStep (e.g. force it to 45 deg. steps) |
// Add a 45° angle layer
ImageEnView1.AddLayer( ielkAngle );
TIEAngleLayer( ImageEnView1.CurrentLayer ).SetPoints([Point(1000, 0), Point(0, 1000), Point(1000, 1000)]);
ImageEnView1.Update();
// which is the same as:
ImageEnView1.AddLayer( ielkAngle );
TIEAngleLayer( ImageEnView1.CurrentLayer ).SetPoints( 0, 1000, 0 );
TIEAngleLayer( ImageEnView1.CurrentLayer ).SetPoints( 1, 0, 1000 );
TIEAngleLayer( ImageEnView1.CurrentLayer ).SetPoints( 2, 1000, 1000 );
ImageEnView1.Update();
// All of the following produce an angle similar to this
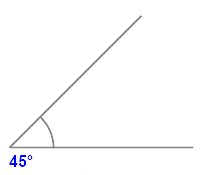
xx := 100;
yy := 100;
// SET BY POINTS
ImageEnView1.LayersAdd( ielkAngle );
with TIEAngleLayer( ImageEnView1.CurrentLayer ) do
begin
// Set by points on bitmap
SetPoints( 0, xx + 180, yy + 130, iepbBitmap );
SetPoints( 1, xx + 0 , yy + 130, iepbBitmap );
SetPoints( 2, xx + 130, yy + 0 , iepbBitmap );
// Which is the same as:
// SetPoints( [ Point( xx + 130, yy + 0 ), Point( xx + 0, yy + 130 ), Point( xx + 130, yy + 0 ), iepbBitmap );
end;
ImageEnView1.Update();
// SET BY SIZE
ImageEnView1.LayersAdd( ielkAngle );
with TIEAngleLayer( ImageEnView1.CurrentLayer ) do
begin
PosX := xx;
PosY := yy;
// Note: Size is larger than above because we must take text and arc into account
Width := 209;
Height := 159;
// Set as a percentage (actually range from 0 - 1000) of the width
SetPoints( 0, 1000, 1000 );
SetPoints( 1, 0 , 1000 );
SetPoints( 2, 720 , 0 );
// Which is the same as:
// SetPoints( [ Point( 1000, 1000 ), Point( 0, 1000 ), Point( 720 , 0 ) ], iepbBitmap );
end;
ImageEnView1.Update();
// SET BY ANGLE
ImageEnView1.LayersAdd( ielkAngle );
with TIEAngleLayer( ImageEnView1.CurrentLayer ) do
begin
PosX := xx;
PosY := yy;
// Note: Size is larger than above because we must take text and arc into account
// Even then, result will be different because we do not set the line lengths
Width := 209;
Height := 159;
// Set by angle
StartAngle := 0;
SweepAngle := 45;
end;
ImageEnView1.Update();
See Also
◼GetPoints◼Points