Properties · Methods · Demos · Examples
Declaration
TIEAngleLayer = class(TIELayer);
Description
TImageEnView supports multiple layers, allowing the creation of a single image from multiple source images (which can be resized, rotated, moved, etc).
TIEAngleLayer is a descendent of
TIELayer that displays an angle formed from three points, and a label showing the angle value.
You can create angle layers with code using
LayersAdd or by user action by setting
MouseInteractLayers:
Item | Description |
mlClickCreateAngleLayers | Click three points to create an angle layer |
mlEditLayerPoints | Click and drag points to move them |
mlCreateAngleLayers | Drag the area of an angle layer. Include mlRotateLayers to rotate the sweep angle (Hold Ctrl to adjust start angle instead) |
Review the
Layer Documentation for a full description of layer support.
Demo | Description | Demo Project Folder | Compiled Demo |
Line Layer Editing | Creating and point editing line, poly-line and angle layers | LayerEditing\Layers_Lines\Layers.dpr | |
All Layer Editing | Usage of image, shape, text, polygon and line layers | LayerEditing\Layers_AllTypes\Layers.dpr | |
// All of the following produce an angle similar to this
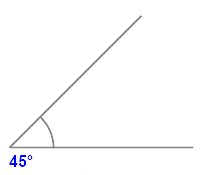
xx := 100;
yy := 100;
// SET BY POINTS
ImageEnView1.LayersAdd( ielkAngle );
with TIEAngleLayer( ImageEnView1.CurrentLayer ) do
begin
LineWidth := 2;
LineColor := clDkGray;
LabelFont.Color := clBlue;
LabelFont.Style := [fsBold];
// Set by points on bitmap
SetPoints( 0, xx + 180, yy + 130, iepbBitmap );
SetPoints( 1, xx + 0 , yy + 130, iepbBitmap );
SetPoints( 2, xx + 130, yy + 0 , iepbBitmap );
// Which is the same as:
// SetPoints( [ Point( xx + 130, yy + 0 ), Point( xx + 0, yy + 130 ), Point( xx + 130, yy + 0 ), iepbBitmap );
end;
ImageEnView1.Update();
// SET BY SIZE
ImageEnView1.LayersAdd( ielkAngle );
with TIEAngleLayer( ImageEnView1.CurrentLayer ) do
begin
PosX := xx;
PosY := yy;
// Note: Size is larger than above because we must take text and arc into account
Width := 209;
Height := 159;
LineWidth := 2;
LineColor := clDkGray;
LabelFont.Color := clBlue;
LabelFont.Style := [fsBold];
// Set as a percentage (actually range from 0 - 1000) of the width
SetPoints( 0, 1000, 1000 );
SetPoints( 1, 0 , 1000 );
SetPoints( 2, 720 , 0 );
// Which is the same as:
// SetPoints( [ Point( 1000, 1000 ), Point( 0, 1000 ), Point( 720 , 0 ) ], iepbBitmap );
end;
ImageEnView1.Update();
// SET BY ANGLE
ImageEnView1.LayersAdd( ielkAngle );
with TIEAngleLayer( ImageEnView1.CurrentLayer ) do
begin
PosX := xx;
PosY := yy;
// Note: Size is larger than above because we must take text and arc into account
// Even then, result will be different because we do not set the line lengths
Width := 209;
Height := 159;
LineWidth := 2;
LineColor := clDkGray;
LabelFont.Color := clBlue;
LabelFont.Style := [fsBold];
// Set by angle
StartAngle := 0;
SweepAngle := 45;
end;
ImageEnView1.Update();
General
Style
Size and Position
Unique to TIEAngleLayer
See Also
◼Layer Editing Overview
◼LayersAdd
◼LayersInsert
◼AdvancedDrawAngle