TIECanvas.AdvancedDrawLine
Declaration
// Standard overload
function AdvancedDrawLine(X1, Y1, X2, Y2 : Integer;
LineColor: TColor;
LineWidth: Integer;
LineStyle: TIEGPPenStyle;
StartingShape, EndingShape: TIELineEndShape;
ShapeSize: Integer;
ShapeFillColor: TColor = clWhite; // Shape uses LineColor and LineWidth for Pen
LineCurve: Double = 0;
AntiAlias: Boolean = True;
CalculateOnly: Boolean = False
): TRect; overload;
// Text Label overload
function AdvancedDrawLine(X1, Y1, X2, Y2 : Integer;
LineColor: TColor;
LineWidth: Integer;
LineStyle: TIEGPPenStyle;
StartingShape, EndingShape: TIELineEndShape;
ShapeSize: Integer;
ShapeFillColor: TColor; // Shape uses LineColor and LineWidth for Pen
// Text Label
LabelPosition: TIELineLabelPos;
const LabelText: String;
LabelFont: TFont;
LabelAutoShrink: Boolean = True;
LabelShape: TIEShape = iesRectangle;
LabelFillColor: TColor = clWhite;
LabelFillColor2: TColor = clBlue;
LabelFillGradient: TIEGDIPlusGradient = gpgNone;
LabelOpacity: Double = 1.0;
LabelHorzMargin: Double = 0;
LabelVertMargin: Double = 0;
// Other parameters
LineCurve: Double = 0;
AntiAlias: Boolean = True;
CalculateOnly: Boolean = False
): TRect; overload;
// Full Overload
function AdvancedDrawLine(X1, Y1, X2, Y2: Integer;
LineColor: TColor;
LineWidth: Integer;
LineStyle: TIEGPPenStyle;
StartingShape, EndingShape: TIELineEndShape;
ShapeSize: Integer;
ShapeFillColor: TColor; // Shape uses LineColor and LineWidth for Pen
// Text Label
LabelPosition: TIELineLabelPos;
const LabelText: String;
LabelFont: TFont;
LabelAutoShrink: Boolean = True;
LabelShape: TIEShape = iesRectangle;
LabelBorderWidth: Integer = 1;
LabelBorderColor: TColor = clBlack;
LabelFillColor: TColor = clWhite;
LabelFillColor2: TColor = clBlue;
LabelFillGradient: TIEGDIPlusGradient = gpgNone;
LabelOpacity: Double = 1.0;
LabelHorzMargin: Double = 0;
LabelVertMargin: Double = 0;
// Advanced Text Styling
TextBorderWidth: Integer = 0;
TextBorderColor: TColor = clBlack;
TextFillTransparency1: Integer = 255;
TextFillTransparency2: Integer = 255;
TextFillColor2: TColor = clRed;
TextFillGradient: TIEGDIPlusGradient = gpgNone;
// Other parameters
LineCurve: Double = 0;
AntiAlias: Boolean = True;
CalculateOnly: Boolean = False
): TRect; overload;
Description
Draws a line that starts at X1,Y1 and ends at X2,Y2. It can optionally include:
◼Start and ending shapes (e.g. arrows)
◼Label with text
For a simpler method, use:
DrawLine.
General Properties
Parameter | Description |
X1, Y1 | The starting point of the line. Note: This ignores any end shapes and labels |
X2, Y2 | The ending point of the line. Note: This ignores any end shapes and labels |
LineColor | Color of the line |
LineWidth | Width of the line |
LineStyle | How the line is drawn |
StartingShape, EndingShape | The shape of any objects at the start/end of the line |
ShapeSize | Size of the start/end shapes |
ShapeSize | Fill color of the start/end shapes (LineColor and LineWidth are used for the border) |
Text Label
Parameter | Description |
LabelPosition | Where the text label is drawn on the line |
LabelText | The string to output to the label |
LabelFont | Optional: The font for the text. You can create a temporary TFont object, or specify NIL to use the Canvas.Font |
LabelAutoShrink | If True, and the text is too large for the label, the font size will be reduced until it fits |
LabelShape | The shape of frame |
LabelBorderColor | The color of the label border (or clNone for no border) |
LabelBorderWidth | The width of the label border |
LabelFillColor | The color of the label fill (or clNone for no fill) |
LabelFillColor2 | The color of the secondary fill (if the label fill has a gradient) |
LabelFillGradient | The direction of the gradient for the label fill (from LabelFillColor to LabelFillColor2). gpgNone specifies no gradient |
LabelOpacity | Specifies the opacity of the label (from 0.0 to 1.0) |
LabelHorzMargin, LabelVertMargin | Adds margin to the label |
Advanced Text Styling
Parameter | Description |
TextBorderWidth | If not 0 the text will have a colored border of the specified width |
TextBorderColor | The color of the text border |
TextFillTransparency1 | The transparency of the text fill (the color is specified by Font.Color) |
TextFillTransparency2 | The transparency of the secondary fill (if the text fill has a gradient) |
TextFillColor2 | The color of the secondary fill (if the text fill has a gradient). The fill color is specified by Font.Color. gpgNone specifies no gradient |
TextFillGradient | The direction of the gradient for the text fill (from Font.Color to TextFillColor2) |
Other Parameters
Parameter | Description |
LineCurve | Specifies the curvature of the line as a multiplier to increase or decrease the radius of the curve. 0: No curve, 1: Curve of an exact half circle, >1 creates a shallower curve, <1 creates a bulging curve. Pass as negative to sweep clockwise |
AntiAlias | True uses best quality. False disables anti-aliasing |
CalculateOnly | If true, the text is not drawn, just the output size returned as the result |
Result is the size of the entire draw in pixels (i.e. it may be outside the passed line points if a label or ending shape has been specified).
| Demos\ImageEditing\EveryMethod\EveryMethod.dpr |
// Draw a pointer
ImageEnView1.IEBitmap.IECanvas.AdvancedDrawLine( 40, 30, 200, 100,
clBlack, 2,
ieesArrow, ieesCircle,
20, clRed );
ImageEnView1.Update();
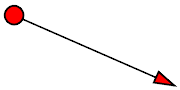
// Draw a line pointer with a label
ImageEnView1.IEBitmap.IECanvas.Font.Name := 'Tahoma';
ImageEnView1.IEBitmap.IECanvas.Font.Size := 8;
ImageEnView1.IEBitmap.IECanvas.Font.Style := [fsBold];
ImageEnView1.IEBitmap.IECanvas.Font.Color := clBlack;
ImageEnView1.IEBitmap.IECanvas.AdvancedDrawLine( 140, 30, 240, 100,
clBlack, 2,
ieesArrow, ieesNone,
20, clRed,
ielpAtEnd, 'Labeled Pointer',
nil, True,
iesRectangle, clRed, clYellow,
gpgVertical );
ImageEnView1.Update();
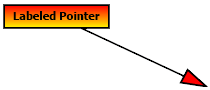
See Also
◼DrawLine
◼LineTo
◼AdvancedDrawAngle
◼AdvancedDrawPolyline
◼AdvancedDrawShape
◼AdvancedDrawText