ImageEn, unit imageenproc |
|
TImageEnProc.CreateTransitionBitmap
Declaration
procedure CreateTransitionBitmap(TransitionProgress: Single; DestBitmap: TIEBitmap);
Description
Use with
PrepareTransitionBitmaps to create a series of frames that transition from one bitmap to another.
Parameter | Description |
TransitionProgress | The percentage that it is has progressed from the start image to the end image (ranging from 0.0 to 100.0) |
DestBitmap | Will be filled with the created transition frame. It must be created before calling and will be automatically sized and set to 24 bit |
Note:
◼This is only for creating transition bitmaps for external purposes. To show an image with a transition effect in ImageEn, use
RunTransition◼If DestBitmap is attached to a
TImageEnView, it will automatically call
Update | Demos\Multi\CreateTransitionFrames\CreateTransitionFrames.dpr |
| Demos\ImageEditing\EveryMethod\EveryMethod.dpr |
procedure TransitionFrameCreationExample();
var
OldBitmap, NewBitmap, TransBitmap: TBitmap;
i: Integer;
TransLevel: Single;
begin
OldBitmap := TBitmap.Create;
NewBitmap := TBitmap.Create;
TransBitmap := TBitmap.Create;
try
OldBitmap.LoadFromFile('C:\OldImage.bmp');
NewBitmap.LoadFromFile('C:\NewImage.bmp');
// Call PrepareTransitionBitmaps once
ImageEnProc.PrepareTransitionBitmaps(OldBitmap, NewBitmap, iettCrossDissolve);
for i := 1 to 9 do
begin
// Transition levels from 10% to 90%
TransLevel := i * 10;
// Call CreateTransitionBitmap for each required frame
ImageEnProc.CreateTransitionBitmap(TransLevel, TransBitmap);
TransBitmap.SaveToFile('C:\TransImage' + IntToStr(I) + '.bmp');
end;
finally
OldBitmap.Free;
NewBitmap.Free;
TransBitmap.Free;
end;
end;
// Random transition from fully black to an image
bmpOut := TIEBitmap.Create( 'D:\TestImage.jpg' );
bmpIn := TIEBitmap.Create( bmpOut.Width, bmpOut.Height, clBlack );
// Prepare transition
trans := TIETransitionType( 1 + Random( ord( High( TIETransitionType )) - 2 )); // Random Transition
ImageEnView1.Proc.PrepareTransitionBitmaps( bmpIn, bmpOut, trans );
// Get transition image
ImageEnView1.Proc.CreateTransitionBitmap( percentage, ImageEnView1.IEBitmap );
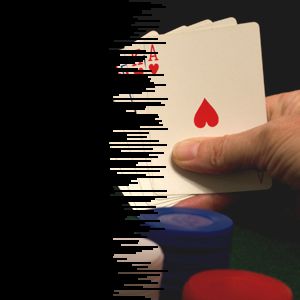
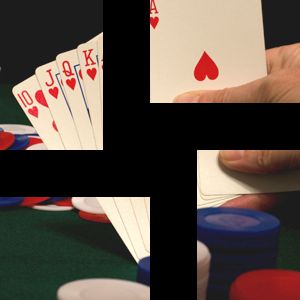
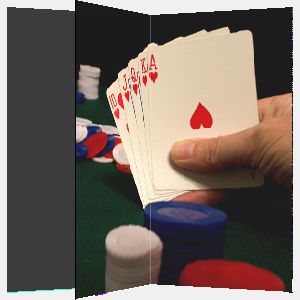
// Create an AVI transitioning one image to another
const
Frames_Per_Second = 20;
Display_Seconds = 5;
var
proc: TImageEnProc;
io: TImageEnIO;
startBitmap, endBitmap : TIEBitmap;
i, frameCount: Integer;
transLevel: Single;
begin
startBitmap := TIEBitmap.Create;
endBitmap := TIEBitmap.Create;
proc := TImageEnProc.Create( nil );
io := TImageEnIO.Create( nil );
try
if io.CreateAVIFile('D:\Transition.avi', Frames_Per_Second, 'cvid' ) = ieaviNOCOMPRESSOR then
raise Exception.create( 'This compressor is unavailable!' );
startBitmap.LoadFromFile( 'D:\image1.jpg' );
endBitmap.LoadFromFile( 'D:\image2.jpg' );
// Call PrepareTransitionBitmaps once
proc.PrepareTransitionBitmaps(startBitmap, endBitmap, SelectedTransitionEffect);
frameCount := Display_Seconds * Frames_Per_Second;
for i := 0 to frameCount - 1 do
begin
// We want levels from 0% to 100% (show start and end)
transLevel := 100 / ( frameCount - 1 ) * i;
// Call CreateTransitionBitmap for each required frame
proc.CreateTransitionBitmap( transLevel, io.IEBitmap );
io.SaveToAVI();
Caption := IntToStr( Round( i / frameCount * 100 )) + '%';
end;
io.CloseAVIFile();
Caption := 'Done!';
finally
startBitmap.Free();
endBitmap.Free();
proc.Free();
io.Free();
end;
end;
See Also
◼PrepareTransitionBitmaps