ImageEn, unit imageenview |
|
TImageEnView.RunTransition
Declaration
// Regular transition overloads
procedure RunTransition(Effect: TIETransitionType; Duration: integer = -1); overload;
// Pan-Zoom transition overloads
procedure RunTransition(PanZoomEffect: TIEPanZoomType; EffectZoom: Integer = 20; Duration: integer = -1; Smoothing: Integer = 96); overload;
procedure RunTransition(StartRect, EndRect: TRect; MaintainAspectRatio: Boolean = True; Duration: integer = -1; Smoothing: Integer = 96); overload;
// Full overloads
procedure RunTransition(Effect: TIETransitionType; Duration: integer; PanZoomEffect: TIEPanZoomType; EffectZoom: Integer; Smoothing: Integer = 96); overload;
procedure RunTransition(Effect: TIETransitionType; Duration: integer; StartRect, EndRect: TRect; MaintainAspectRatio: Boolean; Smoothing: Integer = 96); overload;
Description
RunTransition starts the transition using
Effect and
Duration parameters.
Effect specifies the effect. Duration specifies the display time of the transition in milliseconds (if -1 is specifed the
default duration is used).
If effect is iettPanZoom then use one of the overloaded versions:
Pan-Zoom Overload 1:
Value | Description |
PanZoomEffect | One of ImageEn's built-in Pan-Zoom effects |
EffectZoom | If the specified PanZoomEffect is a "Zoom" type, then this specifies the maximum amount to zoom in, e.g. 20% |
Smoothing | In order to reduce the "jumpiness" of pan-zoom effects, transition frames are alpha blended. A low value will improve smoothness, but increase blurriness. A high value will improve clarity, but increase jumpiness. Typical range is 64 - 196. 255 means no alpha blending |
Pan-Zoom Overload 2:
Value | Description |
StartRect | The starting rectangle of an iettPanZoom transition (specified in bitmap points) |
EndRect | The ending rectangle of an iettPanZoom transition (specified in bitmap points) |
MaintainAspectRatio | StartRect and EndRect will be automatically adjusted to ensure the image appears with the correct aspect ratio |
Smoothing | In order to reduce the "jumpiness" of pan-zoom effects, transition frames are alpha blended. A low value will improve smoothness, but increase blurriness. A high value will improve clarity, but increase jumpiness. Typical range is 64 - 196. 255 means no alpha blending |
iettCubeRotateFromLeft2
iettShreddedFromLeft
iettRandomBoxesWithWord
Note:
◼An easier way to use display transition is just to set
TransitionEffect
◼If the transition effect is not a Pan-Zoom (iettPanZoom) transition, then you must call PrepareTransition() before calling RunTransition()
| Demos\Display\Transitions\Transitions.dpr |
| Demos\Display\PanZoomEffects\PanZoomEffects.dpr |
// Transition from Image1.jpg to Image2.jpg with "Random Points" transition
ImageEnView1.IO.LoadFromFile('D:\image1.jpg'); // Load initial image
ImageEnView1.PrepareTransition(); // Prepare the transition
ImageEnView1.IO.LoadFromFile('D:\image2.jpg'); // Load the next image (but does not display it)
ImageEnView1.RunTransition(iettRandompoints, 500); // Execute the transition
// Automatically adjust starting and ending rect to ensure the transition does not distort the image
// Pan from the top-left corner of the image to the bottom-right
ImageEnView1.IO.LoadFromFile('D:\image.jpg');
ImageEnView1.RunTransition(iepzPanTopLeftToBottomRight,
20, // 20% zoom effect
2000); // 2 second transition
// Pan from the top-left corner of the image to the bottom-right (Image is 600 x 800)
ImageEnView1.IO.LoadFromFile('D:\image.jpg');
ImageEnView1.RunTransition(Rect(0, 0, 300, 400), // Top-left quarter
Rect(300, 400, 600, 800), // Bottom-right quarter
True,
2000); // 2 second transition
// Set properties for Word transition
ImageEnView1.TransitionParams.WordTransWord := 'BLAM';
ImageEnView1.TransitionParams.WordTransFontName := 'Arial';
ImageEnView1.TransitionParams.WordTransFontStyle := [fsBold];
// Prepare ImageEnView1 for a transition (e.g. so loading the image will not immediately update the display)
ImageEnView1.PrepareTransition();
// Load the next image
ImageEnView1.IO.LoadFromFile( GetNextImage() );
// Run the transition
ImageEnView1.RunTransition( iettRandomBoxesWithWord, 1000 );
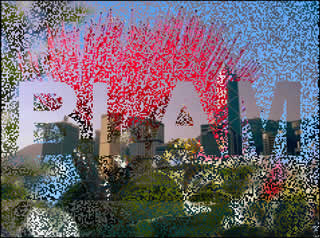
// Slide Transition from old.bmp to new.bmp ensuring that both are shown as the same height
procedure TForm1.btnCompareClick(Sender: TObject);
const
Display_Zoom = 100;
Center_Image = True;
var
bh: Integer;
iz: Double;
begin
// Get original image as transition start
ImageEnView1.IO.LoadFromFile( ExtractFilePath( Application.ExeName ) + 'old.bmp' );
ImageEnView1.Zoom := Display_Zoom;
if Center_Image then
ImageEnView1.CenterImage()
else
ImageEnView1.SetViewXY( 0, 0 );
bh := ImageEnView1.IEBitmap.Height;
iz := ImageEnView1.Zoom;
ImageEnView1.PrepareTransition();
// Load second image as transition result (Zoom to match display size of old image)
ImageEnView1.IO.LoadFromFile( ExtractFilePath( Application.ExeName ) + 'new.bmp' );
ImageEnView1.Zoom := iz / ( ImageEnView1.IEBitmap.Height / bh );
if Center_Image then
ImageEnView1.CenterImage()
else
ImageEnView1.SetViewXY( 0, 0 );
// Run the transition
ImageEnView1.TransitionParams.AlternativeStyle := True; // Include transition line
ImageEnView1.TransitionParams.WipeLineColor := clYellow;
ImageEnView1.RunTransition( iettRightLeft, 3000 ); // Wipe Right to Left
end;
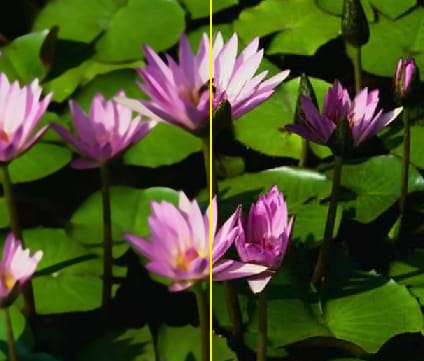
See Also
◼PrepareTransition
◼TransitionRunning
◼AbortTransition