ImageEn, unit imageenproc |
|
TImageEnProc.Blur
Declaration
procedure Blur(radius: Double);
Description
Apply a Gaussian Blur filter of specified radius (> 0) to reduce detail and noise.
Also see:
Comparison of smoothing, blurring and noise reduction methods
Note:
◼A UI for this is available to your users in the
Image Processing dialog
◼Also available as a
RetouchTool by setting
MouseInteractGeneral to [miRetouchTool] and
RetouchMode to iermBlur
◼If the image
PixelFormat is not one of ie24RGB of ie8g, it will be converted
| Demos\ImageEditing\EveryMethod\EveryMethod.dpr |
// Load test image
ImageEnView1.IO.LoadFromFile( 'D:\TestImage.jpg' );
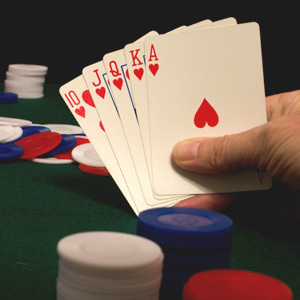
// Apply a Gaussian Blur filter to reduce detail and noise
ImageEnView1.Proc.Blur( 4 );
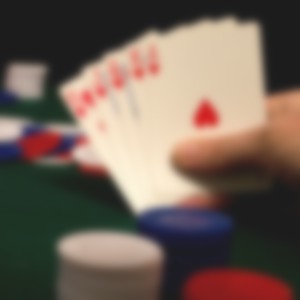
// CREATE BITMAP WITH BLURRED IMAGE FILLING NON-IMAGE AREA
// DestBmp: Output image for result
// BackgroundBmp: Image to use as background (must be valid)
// ImageBmp: Image to draw centered (can be same as BackgroundBmp, or can be NIL)
// Width, Height: Size to make DestBmp
// BlurRadius: How much to blur image (Default is 8, 0 for no blur)
// BackgroundOpacity: If less than 1.0, the background is drawn semi-opaque (with BackgroundColor visible)
procedure StretchDrawWithBlur(DestBmp, BackgroundBmp, ImageBmp: TIEBitmap; Width, Height: Integer; BlurRadius: Integer = 8; BackgroundOpacity: Double = 0.75; BackgroundColor: TColor = clBlack);
var
aRect: TRect;
DestRect : TIERectangle;
begin
// Allocate and size
if not assigned( DestBmp ) then
DestBmp := TIEBitmap.create;
DestBmp.Allocate( Width, Height );
// Fill background color if needed
if BackgroundOpacity < 1.0 then
DestBmp.Fill( BackgroundColor );
// Scale draw the background to fill entire image area
aRect := GetImageRectWithinArea( BackgroundBmp.Width, BackgroundBmp.Height, DestBmp.Width, DestBmp.Height,
0, 0, True, True, True, True, 0,
_fmFillRect_WithOverlap );
DestRect := IERectangle( aRect );
BackgroundBmp.RenderToTIEBitmapEx( DestBmp, DestRect.X, DestRect.Y, DestRect.Width, DestRect.Height,
0, 0, BackgroundBmp.Width, BackgroundBmp.Height,
True, 255, rfNone, ielNormal, BackgroundOpacity );
// Blur the background
if BlurRadius > 0 then
with TImageEnProc.CreateFromBitmap( DestBmp ) do
begin
Blur( BlurRadius );
Free();
end;
// Draw our image
if ImageBmp <> nil then
begin
aRect := GetImageRectWithinArea( ImageBmp.Width, ImageBmp.Height, DestBmp.Width, DestBmp.Height );
DestRect := IERectangle( aRect );
ImageBmp.RenderToTIEBitmapEx( DestBmp, DestRect.X, DestRect.Y, DestRect.Width, DestRect.Height,
0, 0, ImageBmp.Width, ImageBmp.Height,
True, 255, rfNone, ielNormal, 1.0 );
end;
end;
// TEST METHOD
procedure TImageEnViewForm.Button1Click(Sender: TObject);
var
bmpIn, bmpOut: TIEBitmap;
begin
bmpIn := TIEBitmap.Create;
bmpOut := TIEBitmap.Create();
try
bmpIn.Read( 'D:\image.jpeg' );
StretchDrawWithBlur( bmpOut, bmpIn, bmpIn, 900, 400, 8, 0.75, clGray );
ImageEnView1.Assign( bmpOut );
finally
bmpIn.Free;
bmpOut.Free;
end;
end;
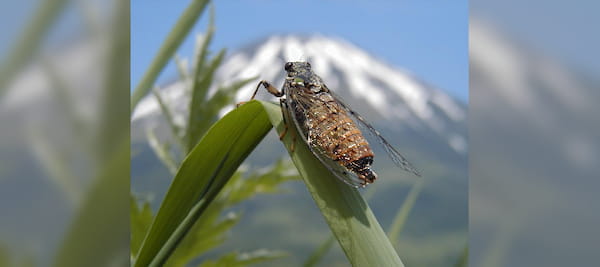