Declaration
property MouseInteractGeneral: TIEMouseInteract;
Description
Specify which mouse activities are performed when the user interacts with the ImageEnView component with the mouse.
Note:
◼Mouse interaction options are split into two properties:
MouseInteractGeneral and
MouseInteractLayers. Items of
MouseInteractLayers relate only to layer editing and creation, whereas
MouseInteractGeneral are all remaining options (selection, view, measurement and image editing functions)
◼Multiple interactions can be specified, but activities that are not mutually compatible will be excluded
◼Setting
MouseInteractGeneral, will also remove incompatible items from
MouseInteractLayers
◼If
PdfViewer is enabled, only the following mouse interactions can be used: miPdfSelectText, miScroll, miZoom, miSmoothZoom, miDblClickZoom, miMovingScroll
◼If not
showing all pages in the
PdfViewer, you can also set selection interactions: miPdfSelectRgn, iSelectZoom, miSelect, miSelectPolygon, miSelectCircle, miSelectMagicWand, miSelectLasso, miSelectChromaKey
Default: []
| Demos\Other\MouseInteract\MouseInteract.dpr |
| Demos\ImageEditing\CompleteEditor\PhotoEn.dpr |
| Demos\Display\SoftPan\SoftPan.dpr |
// Single left click zoom-in image, single right click zoom-out image, click and
// drag scroll the image (if it is bigger than client area).
ImageEnView1.MouseInteractGeneral := [ miZoom, miScroll ];
...Same but with smooth zooming
ImageEnView1.MouseInteractGeneral := [ miSmoothZoom, miScroll ];
...Similar but must double-click to zoom in, or Shift+double-click to zoom out
ImageEnView1.MouseInteractGeneral := [ miDblClickZoom, miScroll ];
// Allow rectangular selections
ImageEnView1.MouseInteractGeneral := [ miSelect ];
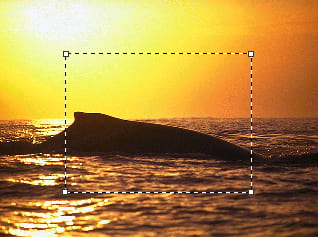
// Allow circular selections
ImageEnView1.MouseInteractGeneral := [ miSelectCircle ];
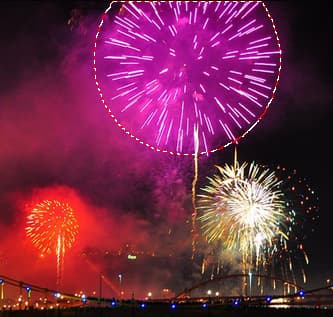
// Allow the user to select the background in an image with a chroma-key background
ImageEnView1.ChromaKeyOptions.Mode := iecSubject;
ImageEnView1.MouseInteractGeneral := [ miSelectChromaKey ];
// Set cursor as an eraser
ImageEnView1.BrushTool.BrushFill := iebfEraser;
ImageEnView1.BrushTool.EraserOpacity := 0.5;
ImageEnView1.MouseInteractGeneral := [ miBrushTool ];
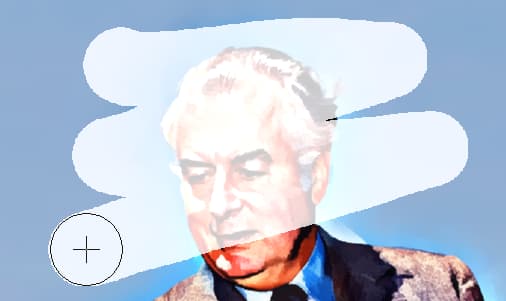
// Use Smart Eraser Brush tool
ImageEnView1.ChromaKeyOptions.Tolerance := 0.15;
ImageEnView1.BrushTool.BrushFill := iebfSmartEraser;
ImageEnView1.MouseInteractGeneral := [ miBrushTool ];
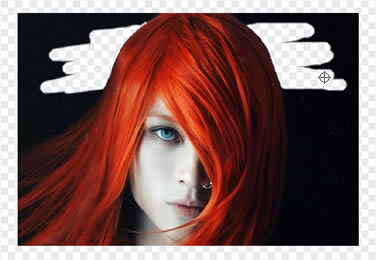
// Smudge the image
ImageEnView1.RetouchTool.RetouchMode := iermSmudge;
ImageEnView1.RetouchTool.SmudgePressure := 15;
ImageEnView1.RetouchTool.Feathering := 3;
ImageEnView1.MouseInteractGeneral := [ miRetouchTool ];
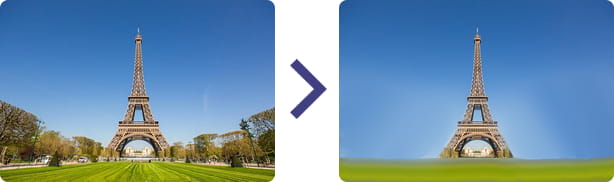
// Apply motion blur to image
ImageEnView1.RetouchTool.RetouchMode := iermMotionBlur ;
ImageEnView1.RetouchTool.MotionBlurSigma := 8;
ImageEnView1.RetouchTool.MotionBlurAngle := 180;
ImageEnView1.RetouchTool.MotionBlurRadius := 10;
ImageEnView1.MouseInteractGeneral := [ miRetouchTool ];
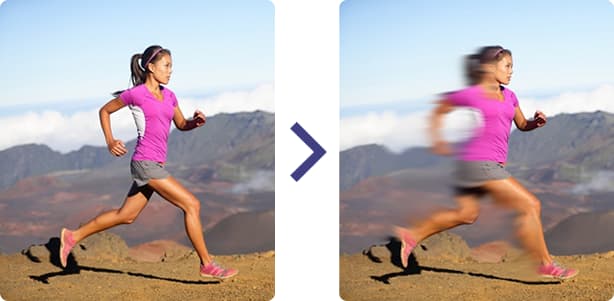
// Use IEVision's Inpainting to remove blemishes in the image
ImageEnView1.RetouchTool.RetouchMode := iermIEVisionInpaint;
ImageEnView1.RetouchTool.InpaintRangeSize := 6;
ImageEnView1.MouseInteractGeneral := [ miRetouchTool ];
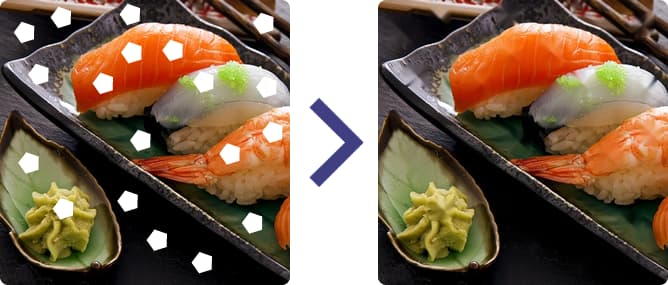
// Allow zooming by selecting the image
ImageEnView1.MouseInteractGeneral := [ miSelectZoom ];
ImageEnView1.DisplayOptions := ImageEnView1.DisplayOptions + [iedoDisableAutoFitWhenZoom];
// Enable measuring of lengths in image (Imperial)
// Set scale of 250 pixels = one inch
ImageEnView1.SetScale( 250, 1, ieuInches );
IEGlobalSettings().DefaultMeasureUnit := ieuInches;
ImageEnView1.Update();
ImageEnView1.MouseInteractGeneral := [ miMeasureLength ];
// Enable measuring of lengths in image (Metric)
// Set 100 pixels = 4cm
ImageEnView1.SetScale( 100, 4, ieuCentimeters );
IEGlobalSettings().DefaultMeasureUnit := ieuCentimeters;
ImageEnView1.Update();
ImageEnView1.MouseInteractGeneral := [ miMeasureLength ];
// Select whole image and allow user to deselect circular regions
With ImageEnView1 do
begin
SelectionBase := iesbBitmap;
Select( 0, 0, IEBitmap.Width, IEBitmap.Height );
ShiftKeyLock := [ iessCtrl_SubFromSel ];
MouseInteractGeneral := [ miSelectCircle ];
end;
// Fill clicked areas with red
ImageEnView1.FillTool.ColorFillValue := clRed;
ImageEnView1.MouseInteractGeneral := [ miColorFill ];
// Fill clicked areas with 50% transparency
ImageEnView1.FillTool.AlphaFillValue := 128;
ImageEnView1.MouseInteractGeneral := [ miAlphaFill ];
// Selecting a color updates all tools
ImageEnView1.FillTool.ColorSelectActions := [ieccChromaKeyColor, ieccBrushColor, ieccFillColor, ieccRotateBackground, ieccLayerFill ];
ImageEnView1.MouseInteractGeneral := [ miColorPicker ];
ImageEnView1.FillTool.ResetAfterColorSelect := True;
// Use miColorPicker to set transparent parts of image
procedure TMainForm.btnSelColorClick(Sender: TObject);
begin
ImageEnView1.MouseInteractGeneral := [ miColorPicker ];
end;
procedure TMainForm.ImageEnView1UserInteraction(Sender: TObject; Event: TIEUserInteractionEvent; Info: Integer);
var
c: TColor;
begin
if Event = ieiColorPickerHover then
begin
// Hovering over image - show color in our panel
pnlCurrentColor.Color := TColor( Info );
end
else
if Event = ieiColorPickerClick then
begin
// Clicked image - set this color as transparent in our image
c := TColor( Info );
ImageEnView1.Proc.SetTransparentColors( c, c, 0);
end;
end;
// Allow user to select images or text and copy to clipboard (automatically detecting selection based on what is under the cursor)
ImageEnView1.MouseInteractGeneral := [ miPdfSelectText, miPdfSelectRgn ];
ImageEnView1.PdfViewer.Enabled := True;
// Allow user to select text and copy to clipboard (image selection disabled)
ImageEnView1.MouseInteractGeneral := [ miPdfSelectText ];
ImageEnView1.PdfViewer.Enabled := True;
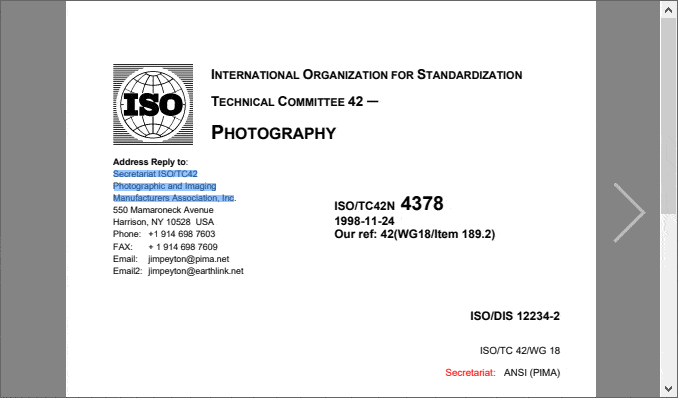
// Allow user to select images and copy to clipboard (text selection disabled)
ImageEnView1.MouseInteractGeneral := [ miPdfSelectRgn ];
ImageEnView1.PdfViewer.Enabled := True;
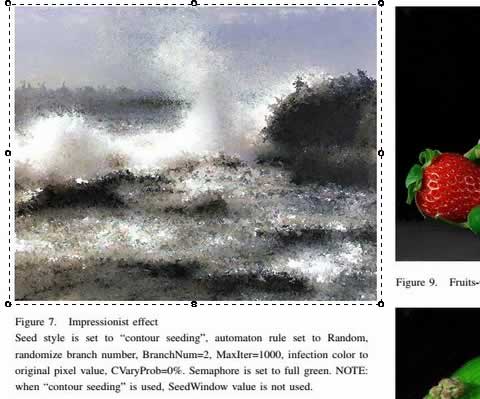
// Display a PDF document and allow editing of the page objects and annotations
ImageEnView1.PdfViewer.Enabled := True;
ImageEnView1.MouseInteractGeneral := [ miPdfSelectObject, miPdfSelectAnnotation ];
ImageEnView1.PdfViewer.AllowObjectEditing := True;
ImageEnView1.IO.LoadFromFilePDF( 'C:\document.pdf' );
Compatibility Information
Prior to v8.6.0, all mouse interactions (both
MouseInteractGeneral and
MouseInteractLayers items) were set with a single property,
MouseInteract. To overcome the size limitation for published sets, this was split into:
◼MouseInteractGeneral: All mouse interactions that are
not related to layer editing
◼MouseInteractLayers: Mouse interactions for layer editing
In most cases, code that was previously:
ImageEnView1.MouseInteract := [ miZoom, miScroll ];
Simply changes to:
ImageEnView1.MouseInteractGeneral := [ miZoom, miScroll ];
However for layer editing items (miMoveLayers, miResizeLayers, miRotateLayers, miCreate*Layers, etc.), the prefix has changed to ml, and you must reference
MouseInteractLayers.
In older versions:
ImageEnView1.MouseInteract := [ miMoveLayers, miResizeLayers ];
Now becomes:
ImageEnView1.MouseInteractLayers := [ mlMoveLayers, mlResizeLayers ];
These are the layer editing items: miMoveLayers, miResizeLayers, miRotateLayers, miCreateImageLayers, miCreateShapeLayers, miCreateLineLayers, miCreatePolylineLayers, miCreateTextLayers, miClickCreateLineLayers, miClickCreatePolylineLayers, miDrawCreatePolylineLayers, miEditLayerPoints
They are now named: mlMoveLayers, mlResizeLayers, mlRotateLayers, mlCreateImageLayers, mlCreateShapeLayers, mlCreateLineLayers, mlCreatePolylineLayers, mlCreateTextLayers, mlClickCreateLineLayers, mlClickCreatePolylineLayers, mlDrawCreatePolylineLayers, mlEditLayerPoints
See Also
◼MouseInteractLayers
◼SelectionOptions
◼OnUserInteraction
◼TIEUserInteraction