Properties · Methods · Demos · Examples
Declaration
TIELineLayer = class(TIELayer);
Description
TImageEnView supports multiple layers, allowing the creation of a single image from multiple source images (which can be resized, rotated, moved, etc).
TIELineLayer is a descendent of
TIELayer that displays a single line. It can optionally display a text label and starting and ending arrows.
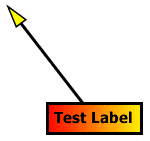
You can create line layers with code using
LayersAdd or by user action by setting
MouseInteractLayers:
Item | Description |
mlClickCreateLineLayers | Click start and end points to draw out a line layer |
mlEditLayerPoints | Click and drag points to move them. Hold Alt key, then click and drag to convert line to a curve |
mlCreateLineLayers | Drag the area of a line layer |
Review the
Layer Documentation for a full description of layer support.
Demo | Description | Demo Project Folder | Compiled Demo |
Line Layer Editing | Creating and point editing line, poly-line and angle layers | LayerEditing\Layers_Lines\Layers.dpr | |
All Layer Editing | Usage of image, shape, text, polygon and line layers | LayerEditing\Layers_AllTypes\Layers.dpr | |
Stamp Layers | Add text, shapes, images and arrows directly to an image by clicking and dragging | LayerEditing\StampTextAndShapes\StampLayers.dpr | |
// Add a layer with yellow filled arrows and circle ends
ImageEnView1.LayersAdd( ielkLine );
TIELineLayer( ImageEnView1.CurrentLayer ).StartShape := ieesArrow;
TIELineLayer( ImageEnView1.CurrentLayer ).EndShape := ieesCircle;
TIELineLayer( ImageEnView1.CurrentLayer ).FillColor := clYellow;
TIELineLayer( ImageEnView1.CurrentLayer ).ShapeSize := 25;
ImageEnView1.Update();
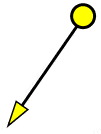
// Hide labels of all line layers when user clicks a check box
procedure Tfmain.chkShowLabelsClick(Sender: TObject);
var
I: integer;
begin
ImageEnView1.LockUpdate();
for I := 0 to ImageEnView1.LayersCount - 1 do
if ImageEnView1.Layers[ I ].Kind = ielkLine then
begin
if chkShowLabels.Checked then
TIELineLayer( ImageEnView1.Layers[ I ]).LabelPosition := ielpAtEnd
else
TIELineLayer( ImageEnView1.Layers[ I ]).LabelPosition := ielpHide;
end;
ImageEnView1.UnlockUpdate();
end;
// Allow users to create and edit line layers
ImageEnView1.MouseInteractLayers := [ mlClickCreateLineLayers, mlEditLayerPoints ];
// Draw a Smiley Face
// make layer lines thicker
ImageEnView1.LayerDefaults.Values[ IELP_BorderWidth ] := '10';
ImageEnView1.LayerDefaults.Values[ IELP_FillColor ] := 'clWhite';
// LEFT EYE
// Add an ellipse shape layer
ImageEnView1.LayersAdd( iesEllipse, 100, 100, 30, 30 );
// RIGHT EYE
// Add an ellipse shape layer
ImageEnView1.LayersAdd( iesEllipse, 170, 100, 30, 30 );
// SMILE
// Add a line layer
ImageEnView1.LayersAdd( Point( 100, 150 ), Point( 200, 150 ));
// Curve it
TIELineLayer(ImageEnView1.CurrentLayer).Curve := 1;
// Show changes
ImageEnView1.Update();
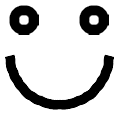
GeneralStyleSize and PositionMeasurement
Unique to TIELineLayerSee Also
◼Layer Editing Overview◼LayersAdd◼LayersInsert◼MouseInteractLayers◼AdvancedDrawLine